If you're looking into and trying out → the new Next.js 13, you might have noticed @next/font →. If you haven't, it's a way to optimize local and external fonts in Next via various advanced techniques. The good thing is they did it for us, and it works!
But as I started thinking about trying it out, I noticed that the docs didn't provide any guidance on how to integrate with Tailwind yet, which I use for my site. I realised that I needed to do some tweaking to make it works smoothly. Without further ado, here's what I did.
Edit: Since writing this, I found out that the same setup also works well with the ./pages
directory. I have added a section on how to use that below.
Previous setup
My previous setup defined fonts like this:
This way, my base font was Inter
, and I used font-heading
and font-subheading
in my className
s wherever I wanted something else. The setup was likely far from optimal, but it worked.
Enter @next/font
The first step I did was to install the package:
Since I was using both local font files and Inter Google Fonts, I imported @next/font/google
and @next/font/local
in my root layout.tsx
file in the ./app
folder.
Setting up layout.tsx
Proceeding in the same file, I defined my three fonts. Note here, that the two local fonts have a variable
defined, while my base font Inter
does not. This is important for the next steps.
Side note: If you're wondering why the Inter font has subsets: ["latin"]
defined, it's to only load a smaller part of the glyphs in the font when all of them are not used. The Next.js docs provide more information here →.
Moving on to the layout definition, here's where things get interesting. I have taken many classNames and combined them into one.
I already mentioned that my base font is Inter. That one I apply using inter.className
. This makes all of the text on the page default to Inter
.
Continuing, the other ones I add using madeDillan.variable
and spaceText.variable
. That way, I can use them in the next step.
Tweaking the Tailwind config
You can now use the CSS variable defined from layout.tsx
in your tailwind.config.js
like this:
Now in my case, I could remove most things from my global.css
.
That's it! This setup lets me keep all of my components and their styling exactly as they were, while still benefiting from the features from @next/font
.
Bonus: usage with the ./pages
directory.
To be honest, I did not know this was possible, but I found out through Twitter. However, setup seems to be unclear and undocumented, so I figured out how to do the same setup in an app on Next 13 without the ./app
directory.
First, I tried importing Inter from @next/font/google
in _document.tsx
. I thought that was resonable, since I have previously had <link>
tags for loading fonts there. However, that cause this error.
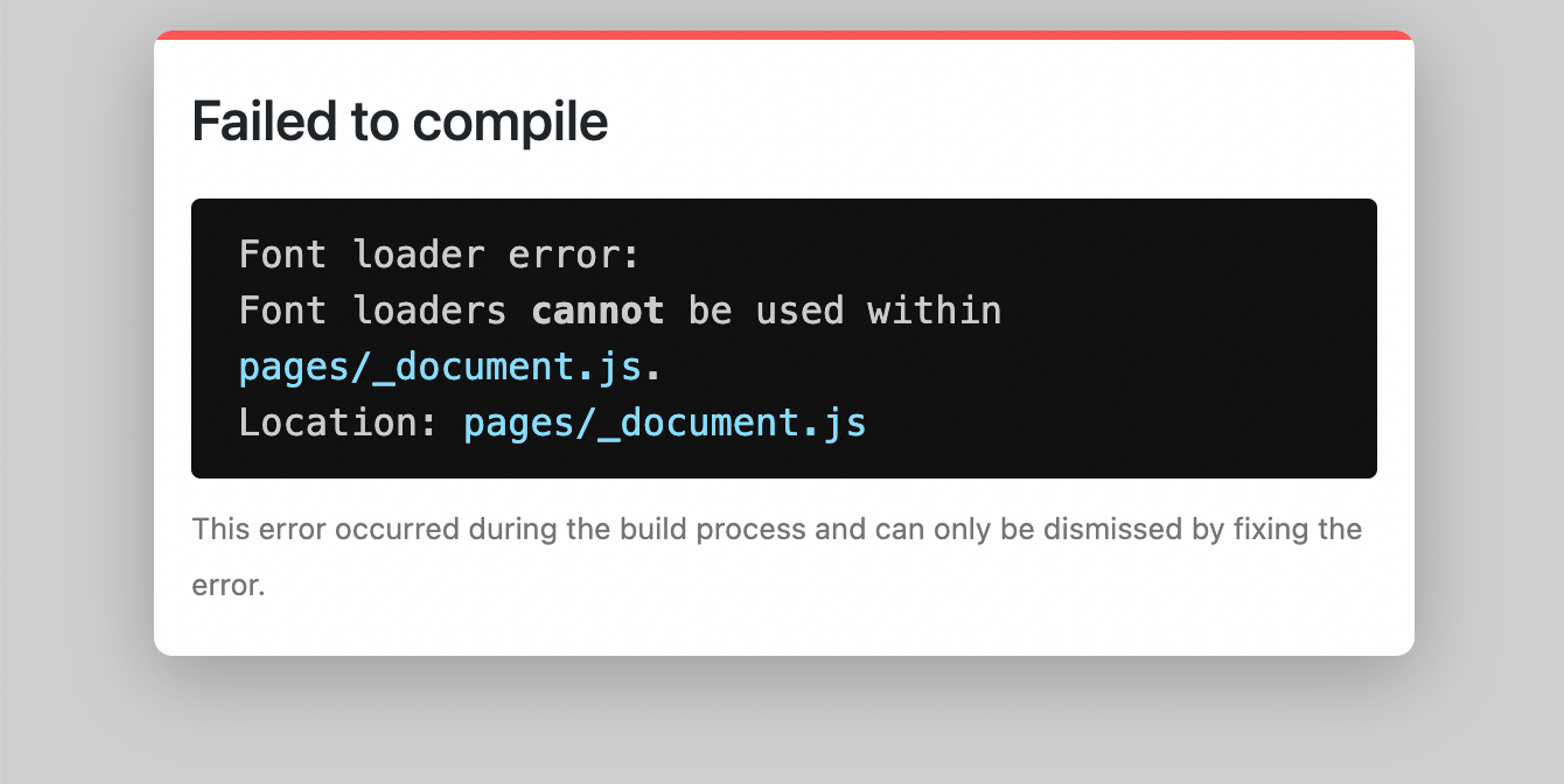
Then I tried implementing something similar to what I did with above but in _app.tsx
. It worked perfectly. Looks something like this:
Hope this helps you try it out!
I'd love to hear your thoughts and feedback here →.